Configuring ESLint and Prettier in Next.js with TypeScript
Learn to install and configure ESLint and Prettier in Next.js with TypeScript to ensure consistent code styling and formatting in your projects.
By Chris Jardine
December 4, 2022
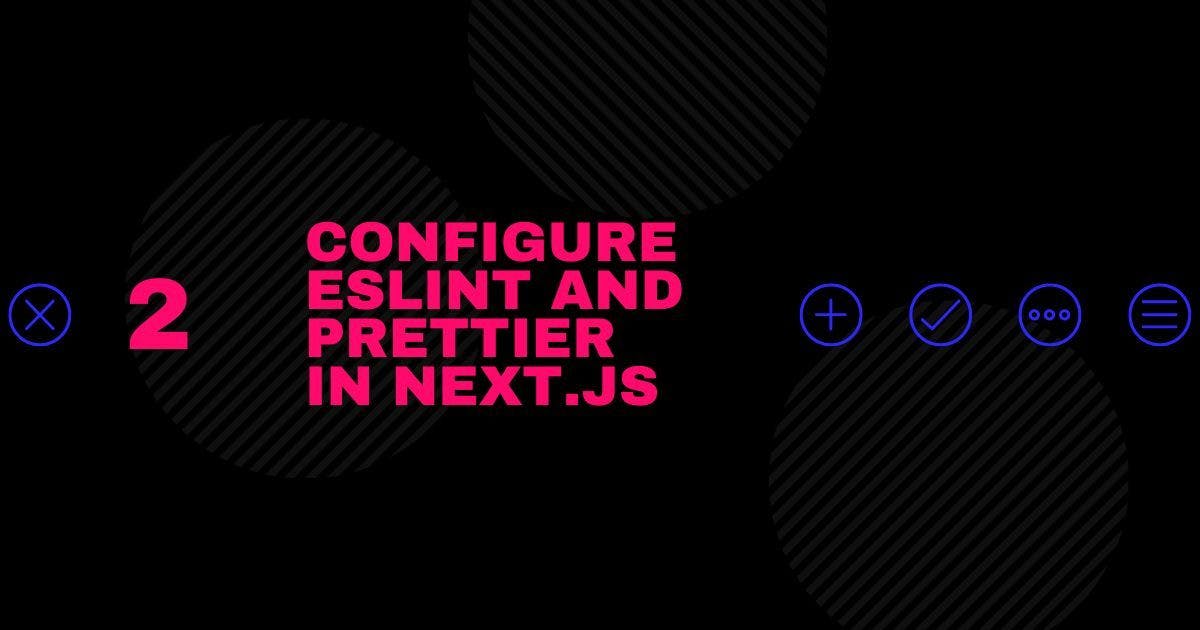
ESLint
ESLint allows us to create a set of rules that define our code style. It statically analyzes our code, informing us of a variety of issues as we're writing code, including syntax errors.
Install ESLint
When creating your Next.js project with npx create-next-app@latest
, select the options to use TypeScript and ESLint. Since version 11, Next.js comes with ESLint preinstalled and preconfigured.
If you’re working on an existing project, install the ESLint plugin for Next.js:
npm i -D @next/eslint-plugin-next
Install TypeScript ESLint
Since we’re using TypeScript, we can add additional, TypeScript-specific, configurations for ESLint. Install the following packages:
npm i -D @typescript-eslint/parser
npm i -D @typescript-eslint/eslint-plugin
Configure ESLint
ESLint comes with a lot of different rules, which you can view here. Additionally, TypeScript ESLint provides us with more rules, which you can view here.
Fortunately, both ESLint and TypeScript ESLint come with recommended configurations that can easily be added to the extends property in your ESLint config file.
.eslintrc.json
{
"extends": [
"next/core-web-vitals",
"eslint:recommended",
"plugin:@typescript-eslint/recommended",
"plugin:@typescript-eslint/recommended-requiring-type-checking",
// ...
],
"plugins": ["@typescript-eslint"]
// ...
}
JSON
Note: "plugin:@typescript-eslint/recommended-requiring-type-checking", isn’t required, but highly recommended. It adds rules that require type information, making our linting process much more robust. It comes at a performance cost, however. But we’re using TypeScript for a reason, and it just makes sense to accept the penalty. You can read more about that here.
Configure TypeScript ESLint
Assuming you’re extending "plugin:@typescript-eslint/recommended-requiring-type-checking", which you should be, you need to make a couple additional changes to your ESLint config. Add the following properties:
.eslintrc.json
{
// ...
"parser": "@typescript-eslint/parser",
"parserOptions": {
"tsconfigRootDir": "./"
"project": ["tsconfig.json"]
}
// ...
}
JSON
First, we set the ESLint parser to the parser used by TypeScript ESLint. Then we told the parser where to find our "tsconfig" file. Make sure to update "parserOptions" if your "tsconfig" file is located elsewhere or has a different extension.
If you don’t add this additional configuration, you won’t see your linting-related warnings and errors in your files. Additionally, trying to lint your project will result in the following error:Error while loading rule '@typescript-eslint/await-thenable': You have used a rule which requires parserServices to be generated. You must therefore provide a value for the "parserOptions.project" property for @typescript-eslint/parser.
Additional configuration
This step is optional, but highly recommended. Add the following rules to the "rules" property in your ESLint config file:
.eslintrc.json
{
// ...
"rules": {
"@typescript-eslint/no-unused-vars": "error",
"@typescript-eslint/no-explicit-any": "error"
// ...
}
}
JSON
The recommended configuration already includes these two rules, but they’re set to "warn". Setting them to "error" will prevent us from building our project. The "no-unused-vars" rule refers to variables the have been declared but not used. Get rid of them if you aren’t using them. The "no-explicit-any" rule refers to anything you use the type "any" on. The "any" type essentially means you don't want to use TypeScript.
Now, let’s move on!
Prettier
Whereas ESLint helps us with code style, it’s not necessarily meant to handle code formatting. This is where we use Prettier. If you haven’t already, install the Prettier VS Code extension.
Configure Prettier
Create a ".prettierrc.json" file at the root of your project. This will contain the Prettier configuration specific to your project. I've included an example configuration below, but additional Prettier options can be found here.
.prettierrc.json
{
"singleQuote": true,
"tabWidth": 2,
"useTabs": false
}
JSON
Install ESLint Config Prettier
In some cases, Prettier rules can conflict with ESLint rules. The "eslint-config-prettier" package can be used to disable these rules:
npm i -D eslint-config-prettier
Add it to the "extends" property in your ESLint config file. Make sure it’s last in the array so it can override the other configurations:
.eslintrc.json
{
"extends": [
// ...,
"prettier"
],
// ...
}
JSON
VS Code
Next, we can set up VS Code to automatically fix our linting and formatting errors.
Configure VS Code's settings for Prettier
Create a file called "settings.json" in a directory called ".vscode" at the root of your project and add the following code:
.vscode/settings.json
{
"editor.defaultFormatter": "esbenp.prettier-vscode",
"editor.formatOnSave": true,
"editor.formatOnPaste": true,
"editor.codeActionsOnSave": {
"source.fixAll.eslint": true,
}
}
JSON
This will set the default formatter to Prettier and run it when you save a file or paste something into it. It also contains an action to fix all auto-fixable ESLint errors on save. Feel free to adjust these options to your liking.
Full Code Examples
Here's a complete example of what your code should look like:
{
"extends": [
"next/core-web-vitals",
"eslint:recommended",
"plugin:@typescript-eslint/recommended",
"plugin:@typescript-eslint/recommended-requiring-type-checking",
"prettier"
],
"root": true,
"parser": "@typescript-eslint/parser",
"parserOptions": {
"project": ["tsconfig.json"]
},
"plugins": ["@typescript-eslint"],
"rules": {
"@typescript-eslint/no-unused-vars": "error",
"@typescript-eslint/no-explicit-any": "error"
}
}
{
"singleQuote": true,
"printWidth": 80,
"tabWidth": 2,
"useTabs": false
}
{
"editor.defaultFormatter": "esbenp.prettier-vscode",
"editor.formatOnSave": true,
"editor.formatOnPaste": true,
"editor.codeActionsOnSave": {
"source.fixAll.eslint": true
},
"files.exclude": {
"**/.git": true,
"**/.svn": true,
"**/.hg": true,
"**/CVS": true,
"**/.DS_Store": true,
"**/Thumbs.db": true,
"**/node_modules": true
}
}
Summary
Good job making it this far—your future self thanks you! We learned how to set up ESLint and TypeScript ESLint in Next.js, which specifies a lot of rules about how our code should be written. We also learned how to set up Prettier to work alongside ESLint to format our code and how to have VS Code perform some of this automatically. With individual configuration files for each, we make sure that anyone working on our project will be forced to use the coding style we want enforced.
More Info
Latest posts
Content modeling with Sanity schemas
January 25, 2023